Last updated December 5th, 2023 22:51
Creating a WordPress user typically happens in two ways. The first is the classic method through the administration interface, and the second, for more experienced users, involves working directly in the database. However, let’s explore a third method that allows you to create a WordPress user very conveniently. In this article, we’ll demonstrate how to create a WordPress admin user using a script and FTP. The principle is simple: we’ll create a PHP file that we’ll easily upload to the website’s FTP. Subsequently, we’ll call it in a browser, and the magic will happen.
The script will generate a user with administrative privileges, show you their password, and then, like in an action movie, delete itself from the FTP. Let’s get into it.
How to Create a WordPress Admin User Using a Script and FTP
What will you need? Not much, just a simple text editor and FTP access to the website. In this specific case, it doesn’t matter whether you access the FTP through an FTP client or via a webFTP service provided by the web host.
The following script performs these actions:
- creates a new WordPress user with the username ‘wpuser’
- assigns administrator rights to this user
- shows you the password generated for this user
- deletes itself from the FTP to erase traces
What’s the purpose of such a script? For instance, in a situation where you’ve forgotten a password, the user’s contact email isn’t functional, and you’re unable to work with the database. The second possibility could arise when you only have FTP access and want to get into WordPress without knowing the access credentials. Certainly, this could be done differently. Via FTP, you’d find the database access, log into phpMyAdmin, and change the hash. However, this method is a bit easier and faster without altering the original website administrator’s password.
Script for creating a user using FTP:
$dash_len) {
$dash_str .= substr($password, 0, $dash_len) . '-';
$password = substr($password, $dash_len);
}
$dash_str .= $password;
return $dash_str;
}
$form = " ";
// Take a array and get random index, same function of array_rand, only diference is
// intent use secure random algoritn on fail use mersene twistter, and on fail use default array_rand
function tweak_array_rand($array){
if (function_exists('random_int')) {
return random_int(0, count($array) - 1);
} elseif(function_exists('mt_rand')) {
return mt_rand(0, count($array) - 1);
} else {
return array_rand($array);
}
}
echo "";
// ----------------------------------------------------
// CONFIG VARIABLES
// Make sure that you set these before running the file.
$newusername = 'wpuser';
$newpassword = generateStrongPassword(24);
$newemail = 'adresa@domena.koncovka';
// ----------------------------------------------------
// This is just a security precaution, to make sure the above "Config Variables"
// have been changed from their default values.
if ( $newpassword != 'YOURPASSWORD' && $newemail != 'YOUREMAIL@TEST.com' && $newusername !='YOURUSERNAME' ) {
// Check that user doesn't already exist
if ( !username_exists($newusername) && !email_exists($newemail) )
{
// Create user and set role to administrator
$user_id = wp_create_user( $newusername, $newpassword, $newemail);
if ( is_int($user_id) )
{
$wp_user_object = new WP_User($user_id);
$wp_user_object->set_role('administrator');
if(is_multisite()) {
grant_super_admin($user_id);
$sites = get_sites();
$user_info = [
"capabilities" => get_user_meta($user_id, "wp_capabilities", true),
"user_level" => get_user_meta($user_id, "wp_user_level", true)
];
foreach($sites as $site) {
update_user_meta($user_id, "wp_" . $site->blog_id . "_capabilities", $user_info['capabilities']);
update_user_meta($user_id, "wp_" . $site->blog_id . "_user_level", $user_info['user_level']);
}
}
echo 'Účet wpuser byl úspěšně vytvořen.
';
echo "Uživatelské jméno
" . $newusername . "
E-mailová adresa" . $newemail . "
";
echo "Heslo" . $newpassword . "";
echo "Aktuální soubor byl smazán.";
unlink(__FILE__);
}
else {
echo 'Během vytváření uživatele wpuser došlo k chybě.';
echo $form;
}
} else {
echo 'Administrátorský účet wpuser již existuje!';
echo $form;
}
}
else {
echo 'Vypadá to, že nebylo vyplněno heslo, uživatelské jméno nebo e-mail. Zkuste to znovu.';
echo $form;
}
if(isset($_POST['removefile'])) {
unlink(__FILE__);
echo "Aktuální soubor byl smazán.";
}
echo "";
What should I do with the script?
First, create a new text document. Copy the PHP code provided above into it. Save the text file and name it user.php. Notice that you’ve changed the file extension from .txt to .php in the filename. That’s fine. Upload this file to the same folder on FTP where you have WordPress installed. Then, execute the file. Type the file’s URL into your browser. In this case, it will be your-domain.extension/user.php.
The script will run, create a user with admin privileges in WordPress, show you the access details, and then delete itself. Now you can use these credentials to log in.
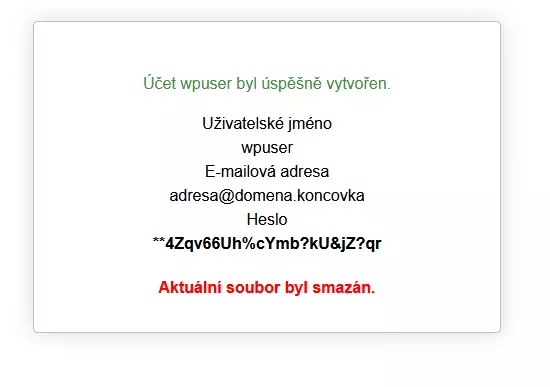
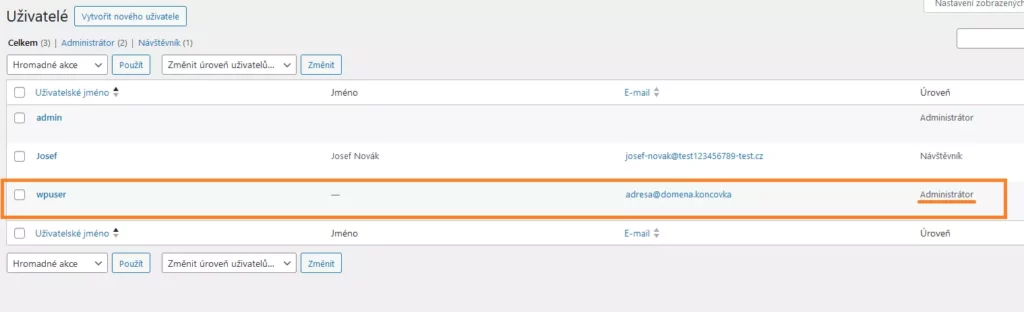
How to create a WordPress admin user using a script and FTP
Conclusion
Use the script judiciously. Its primary function isn’t to infiltrate someone’s editorial system without their knowledge. Essentially, it serves as an emergency system for forgotten passwords, providing an alternative solution.
The website is created with care for the included information. I strive to provide high-quality and useful content that helps or inspires others. If you are satisfied with my work and would like to support me, you can do so through simple options.
Byl pro Vás tento článek užitečný?
Klikni na počet hvězd pro hlasování.
Průměrné hodnocení. 0 / 5. Počet hlasování: 0
Zatím nehodnoceno! Buďte první
Je mi líto, že pro Vás nebyl článek užitečný.
Jak mohu vylepšit článek?
Řekněte mi, jak jej mohu zlepšit.
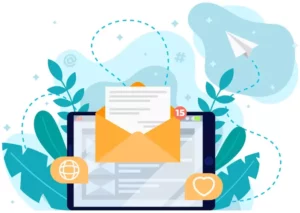
Subscribe to the Newsletter
Stay informed! Join our newsletter subscription and be the first to receive the latest information directly to your email inbox. Follow updates, exclusive events, and inspiring content, all delivered straight to your email.